Debug in preview
❗Warning: This is a legacy page covering functionality with the old SDK version 6. See the latest version of this topic here .
Running a preview provides some useful debugging information and tools to help you understand how the scene is rendered. The preview mode provides indicators that show parcel boundaries and the orientation of the scene.
If the scene can’t be compiled, you’ll just see the grid on the ground, with nothing rendered on it.
If this occurs, there are several places where you can look for error messages to help you understand what went wrong:
- Check your code editor to make sure that it didn’t mark any syntax or logic errors.
- Check the output of the command line where you ran
dcl start
- Check the JavaScript console in the browser for any other error messages. For example, when using Chrome you access this through
View > Developer > JavaScript console
. - If you’re running a preview of a multiplayer scene that runs together with a local server, check the output of the command line window where you run the local server.
If an entity is located or extends beyond the limits of the scene, it will be displayed in red to indicate this, with a red bounding box to mark its boundaries. Nothing in your scene can extend beyond the scene limits. This won’t stop the scene from being rendered locally, but it will stop the offending entities form being rendered in Decentraland.
💡 Tip: Before you deploy your scene to the production environment, deploy it to the test environment to try it in a context that is a lot closer to production. See Development Workflow
Use the console #
Output messages to console (using log()
). You can then view these messages as they are generated by opening the JavaScript console of your browser. For example, when using Chrome you access this through View > Developer > JavaScript console
.
You can also add debugger
commands or use the sources
tab in the developer tools menu to add breakpoints and pause execution while you interact with the scene in real time.
Once you deploy the scene, you won’t be able to see the messages printed to console when you visit the scene in-world. If you need to check these messages on the deployed scene, you can turn the scene’s console messages back on adding the following parameter to the URL: DEBUG_SCENE_LOG
.
Add breakpoints in the Decentraland Editor #
Using the Decentraland Editor, you can add breakpoints to your scene’s code. When running a preview, whenever the code passes through these breakpoints, it pauses execution. A Debug panel opens, showing the current values of all variables at that point in time.
This is especially useful to validate that the data at a given point in time is what you expect. You can also modify the values of any variable manually and resume execution with the blue play button, using those new variables. This is great to test corner cases, to make sure the scene behaves as expected on every scenario, which might otherwise be a lot harder to reproduce.
Make sure you’ve installed the Decentraland editor .
- Open VSCode in a Decentraland scene project.
- Click on the Debugger icon on the left sidebar.
- Click on
Run and Debug
and selectDecentraland
(this step is not necessary if your project already has a.vscode/launch.json
file). - Click on
Run
. A browser should open. - Try setting a breakpoint and interacting with the scene in a way it will step on that line.
View scene stats #
The lower-left corner of the preview informs you of the FPS (Frames Per Second) with which your scene is running. Your scene should be able to run above 25 FPS most of the time.
Click the Y key to open the Panel. This panel displays the following information about the scene, and is updated in real time as things change:
- Processed Messages
- Pending on Queue
- Scene location (preview vs deployed)
- Poly Count
- Textures count
- Materials count
- Entities count
- Meshes count
- Bodies count
- Components count
The processed messages and message queue refer to the messages sent by your scene’s code to the engine. These are useful to know if your scene is running more operations than the engine can support. If many messages get queued up, that’s usually a bad sign.
The other numbers in the panel refer to the usage of resources, in relation to the scene limitations . Keep in mind that the maximum allowed number for these values is proportional to the amount of parcels in the scene. If your scene tries to render an entity that exceeds these values, for example if it has too many triangles, it won’t be rendered in-world once deployed.
📔 Note: Keeping this panel open can negatively impact the frame rate and performance of your scene, so we recommend closing it while not in use.
Run code only in preview #
You can detect if a scene is running as a preview or is already deployed in production, so that the same code behaves differently depending on the case. You can use this to add debugging logic to your code without the risk of forgetting to remove it and having it show in production.
To use this function, import the @decentraland/EnvironmentAPI
library.
import { isPreviewMode } from '@decentraland/EnvironmentAPI'
executeTask(async () => {
const preview: boolean = await isPreviewMode()
if (preview){
log("Running in preview")
}
}
📔 Note: isPreviewMode()
needs to be run as an
async function
, since the response may delay in returning data.
Dependency versions #
Running a Decentraland scene locally depends on two main libraries: decentraland
(the CLI, which is installed globally on your machine) and decentraland-ecs
, which is installed on each project folder. Make sure both of those are up to date, as any issues you’re experiencing might already be fixed in newer versions. There may also be compatibility problems when attempting to run with one of these two outdated and the other up to date. You can run the following commands to update both these libraries to the latest stable version:
npm i -g decentraland@latest
npm i decentraland-ecs@latest
If you’re using any of the
utils libraries
make sure those are also up to date, as older versions of these libraries may not be compatible with newer versions of decentraland-ecs
.
The decentraland-ecs
library has in turn a couple of internal dependencies that are installed with it: the renderer
and the kernel
. Each decentraland-ecs
version is paired with its corresponding versions of both. In occasions, it may be useful to try switching versions of these dependencies independently, to better pinpoint where an issue has originated. You can force your preview to use a different version of the renderer
or of the kernel
by simply providing the url parameters renderer-version
and kernel-version
, pointing at a specific commit.
For example, you can run your preview with the following URL:
http://127.0.0.1:8000/?position=0%2C0&SCENE_DEBUG_PANEL&renderer-version=1.0.12119-20210830195045.commit-a8be53a
To find out what versions are available to choose from on each dependency, check the version history on the NPM pages for the
Renderer
and for the
Kernel
. To know what versions of these dependencies are in use by default by a specific decentraland-ecs
version, you can run the following command, indicating the decentraland-ecs
version you’re curious about:
npm info [email protected]
View bounding boxes #
While running a scene preview, open the debug menu (on the right of the minimap) and click Bounding Boxes to toggle the visualization of bounding boxes on and off.
Bounding boxes are displayed as thin white boxes around each mesh. Bounding boxes show the limits of the space occupied by a 3d model. Every mesh in a 3d model has its own bounding box.
When Decentraland’s engine checks if an entity is within the scene limits, it looks at the positions of each corner of the bounding box. Checking the corners of the bounding boxes is an engine optimization, as checking the position of each vertex in the model would be a lot more work. Ideally the bounding box shouldn’t extend beyond the visible vertexes of the model, but it may not be the case if the model wasn’t carefully built with this in mind.
By visualizing bounding boxes, you can debug problems with entities being reported as outside the scene limits.
Lighting conditions #
The in-world time of day has a big impact on how 3d models look. The color of the light source changes subtly, having a bluish tint at night, and a reddish tint during sunrise and sunset. The direction of the light also moves across the sky, casting shadows in different directions.
Check that your scene looks good at all times of day by switching the game clock to different values. Open the settings panel, and in the General tab set the skybox time to any time you prefer. If this slider is grayed out, make sure that the Dynamic skybox option is disabled.
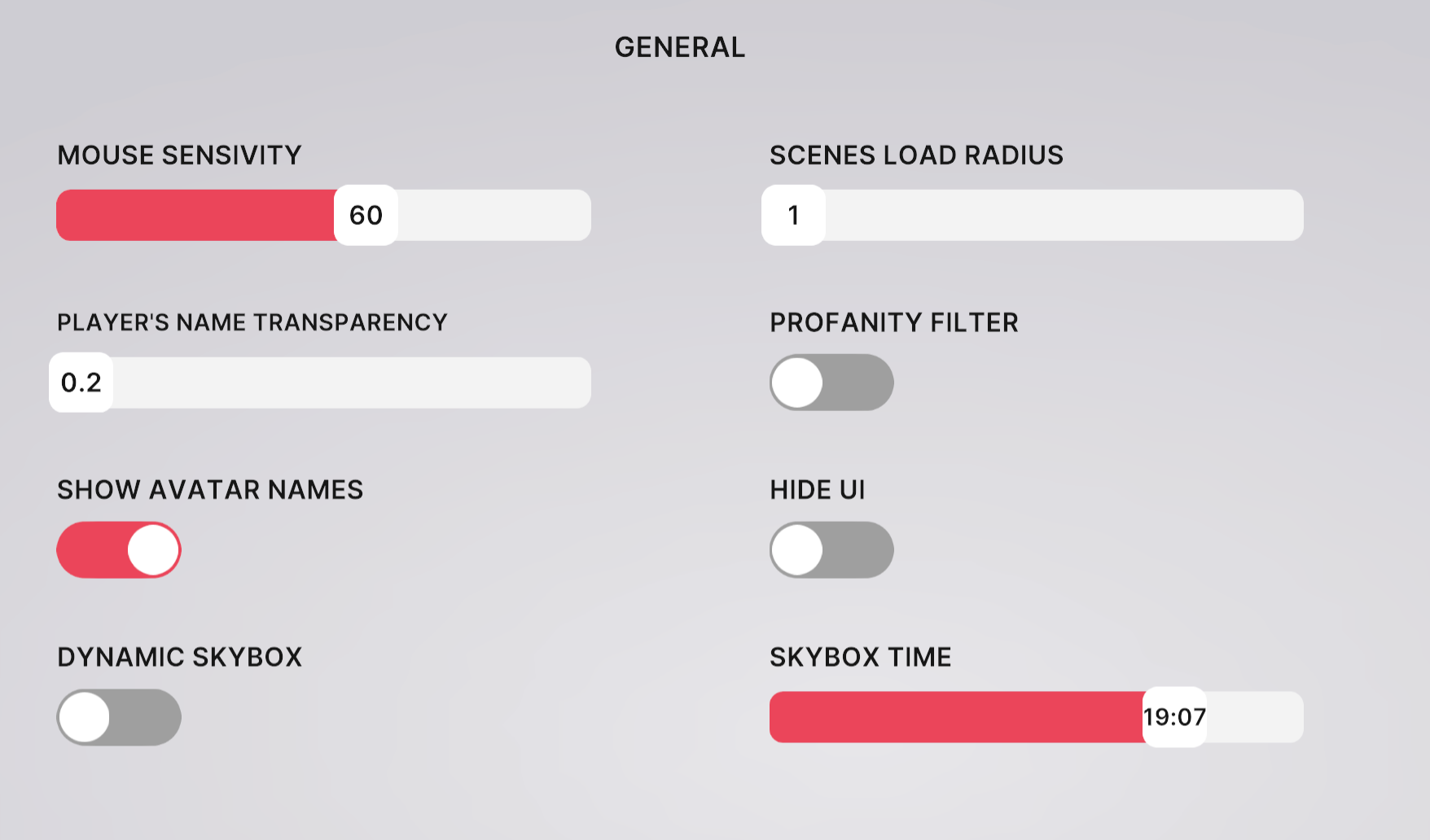
Your 3d model’s materials might not look the same as they did in the modeling tool you created it with. This is to be expected, as all 3d rendering engines have subtle differences in how they deal with light and materials.
Avatars and accounts #
To use the avatar that’s linked to your active Metamask account, with access to all of your owned wearables, you need to run the preview in the browser with Web3.
In the Decentraland Editor, open the Decentraland tab and hover your mouse over it to display the three dots icon on the top-right. Click this icon and select Open in browser with Web3.
With the CLI, you can start the preview with:
dcl start --web3
Alternatively, you can manually add the URL parameter &ENABLE_WEB3
to the URL in the browser window.
When running a preview normally, without a connected Web3 account, you’re assigned a random avatar each time you reload.
To use a consist guest avatar across your sessions, you can store an avatar profile by adding a PLAYER
parameter to the URL with any string as its value. When using this, the preview will store your avatar’s settings locally on your browser, to retrieve them whenever you use the same string on the PLAYER
parameter. For example, every time you open the preview with the URL http://127.0.0.1:8000/?PLAYER=ringo
, you’ll have the same avatar.
Connecting to Ethereum network #
If your scene makes use of transactions over the Ethereum network, for example if it prompts you to pay a sum in MANA to open a door, you need to run the preview in the browser with Web3.
In the Decentraland Editor, open the Decentraland tab and hover your mouse over it to display the three dots icon on the top-right. Click this icon and select Open in browser with Web3.
With the CLI, you can start the preview with:
dcl start --web3
Alternatively, you can manually add the URL parameter &ENABLE_WEB3
to the URL in the browser window.
Using the Ethereum test network #
You can avoid using real currency while previewing the scene. For this, you must use the Ethereum Sepolia test network and transfer fake MANA instead. To use the test network you must set your Metamask Chrome extension to use the Sepolia test network instead of Main network. You must also own MANA in the Sepolia blockchain, which you can acquire for free from Decentraland.
Any transactions that you accept while viewing the scene in this mode will only occur in the test network and not affect the MANA balance in your real wallet.
Multiplayer testing #
If you open a second preview window on your machine, you will enter the scene with a different avatar. The avatars on both tabs will be able to see each other and interact, although currently they might have inconsistent names and wearables on.
📔 Note: You can’t open multiple tabs using the same account. So if your URL has a hardcoded PLAYER
parameter with the same string on multiple tabs, or you’re connecting to Metamask on more than one tab, it won’t be possible to load them all. Each simultaneous tab should load a different account.
If the scene uses the MessageBus to send messages between players, these will work between the different tabs.
If the scene connects to a third party server via websockets, these connections should also work independently on each tab, as separate players.