Display an NFT
❗Warning: This is a legacy page covering functionality with the old SDK version 6. See the latest version of this topic here .
You can display a 2D NFT (Non-Fungible Token) that you own in your Decentraland scenes.
The NTF’s image and other data is taken from an API, based on the token’s contract and id. Any NFTs that are supported on OpenSea can also be displayed in an NFT picture frame in Decentraland.
The picture frame is displayed adjusting to the dimensions of the NFT image. If the image’s dimensions are 512 X 512 pixels, the frame keeps its original size. If the image has different dimensions, the frame will be resized and stretched to match these dimensions.
💡 Tip: If you want to stretch or resize the image from what’s generated by default, you can change thescale
property in the entity’sTransform
component.
Add an NFT #
Add an NFTShape
component to an entity to display a 2D token in your scene.
const entity = new Entity()
const shapeComponent = new NFTShape(
'ethereum://0x06012c8cf97BEaD5deAe237070F9587f8E7A266d/558536'
)
entity.addComponent(shapeComponent)
entity.addComponent(
new Transform({
position: new Vector3(4, 1.5, 4),
})
)
engine.addEntity(entity)
The NFTShape
component must be instanced with a parameter that includes the following:
- The contract of the token (for example, the CryptoKitties contract)
- The id of the specific token you own
The example above fetches an NFT with the contract address 0x06012c8cf97BEaD5deAe237070F9587f8E7A266d
, and the specific identifier 558536
. The corresponding asset asset can be found in OpenSea at
https://opensea.io/assets/0x06012c8cf97BEaD5deAe237070F9587f8E7A266d/558536
.
Customize the frame #
By default, the image will have a purple background and have a frame with a pulsating emissive texture around it. You can set the following properties to better match the style of the NFT and the scene:
color
: Determines the back side of the model, and also the background of the image in case the NFT image has transparency.style
: Selects a frame model from an enum of several predetermined options.
const shapeComponent = new NFTShape(
'ethereum://0x06012c8cf97BEaD5deAe237070F9587f8E7A266d/558536',
{
color: Color3.Green(),
style: PictureFrameStyle.Gold_Edges,
}
)
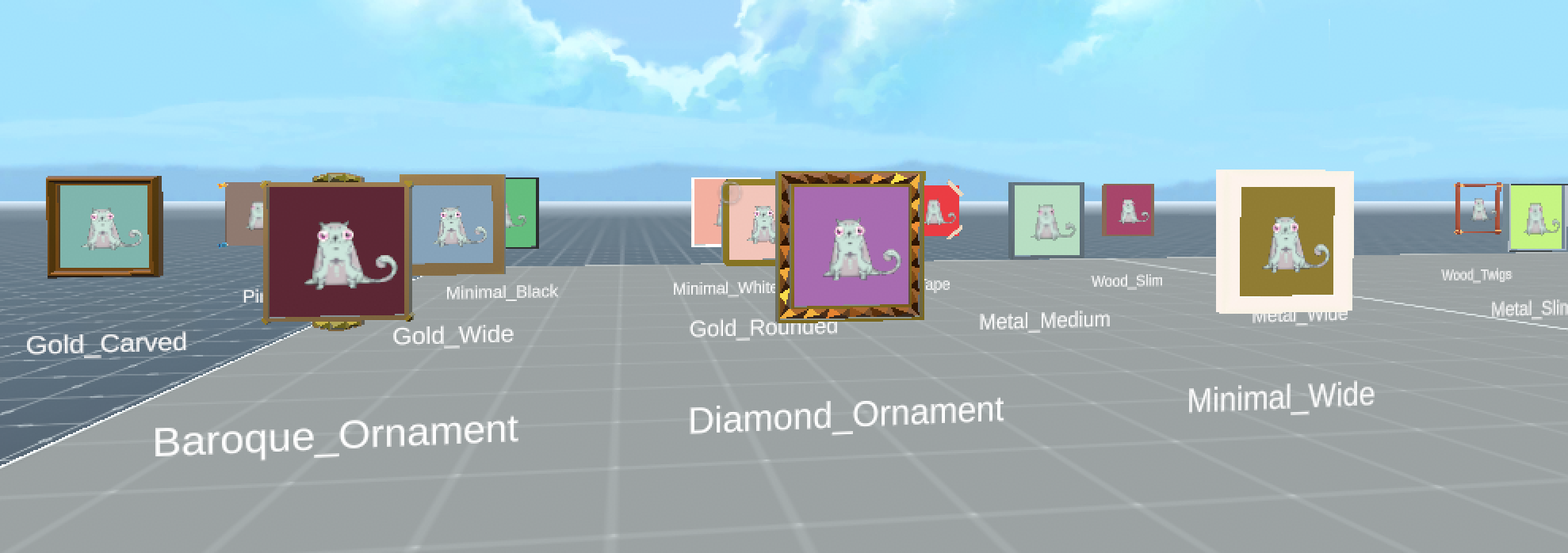
Here’s the full list of supported frame styles:
- Classic
- Baroque_Ornament
- Diamond_Ornament
- Minimal_Wide
- Minimal_Grey
- Blocky
- Gold_Edges
- Gold_Carved
- Gold_Wide
- Gold_Rounded
- Metal_Medium
- Metal_Wide
- Metal_Slim
- Metal_Rounded
- Pins
- Minimal_Black
- Minimal_White
- Tape
- Wood_Slim
- Wood_Wide
- Wood_Twigs
- Canvas
- None
Some frames use more materials than others. For example, the default frame adds 1 material for the NFT itself, 1 material for a background colored plane, and 2 materials for the frame (shared with other picture frames of the same style). If you need to reduce materials to stay within scene limitations , pick a style that is simpler. For example “none” only uses only 1 material for the NFT itself.
💡 Tip: Using Visual Studio Code (or another IDE), see the whole list by typingPictureFrameStyle.
and waiting for the smart suggestions display the list of options. UsePictureFrameStyle.None
to display the plain NFT as is, with no frame or background color.
Open an NFT UI #
Open a prebuilt UI that displays the name, owner, and description of an NFT. It also includes the NFT’s current price and price of last sale if applicable, and a button that links to the NFT’s page on OpenSea, where more information is available and it can be purchased.
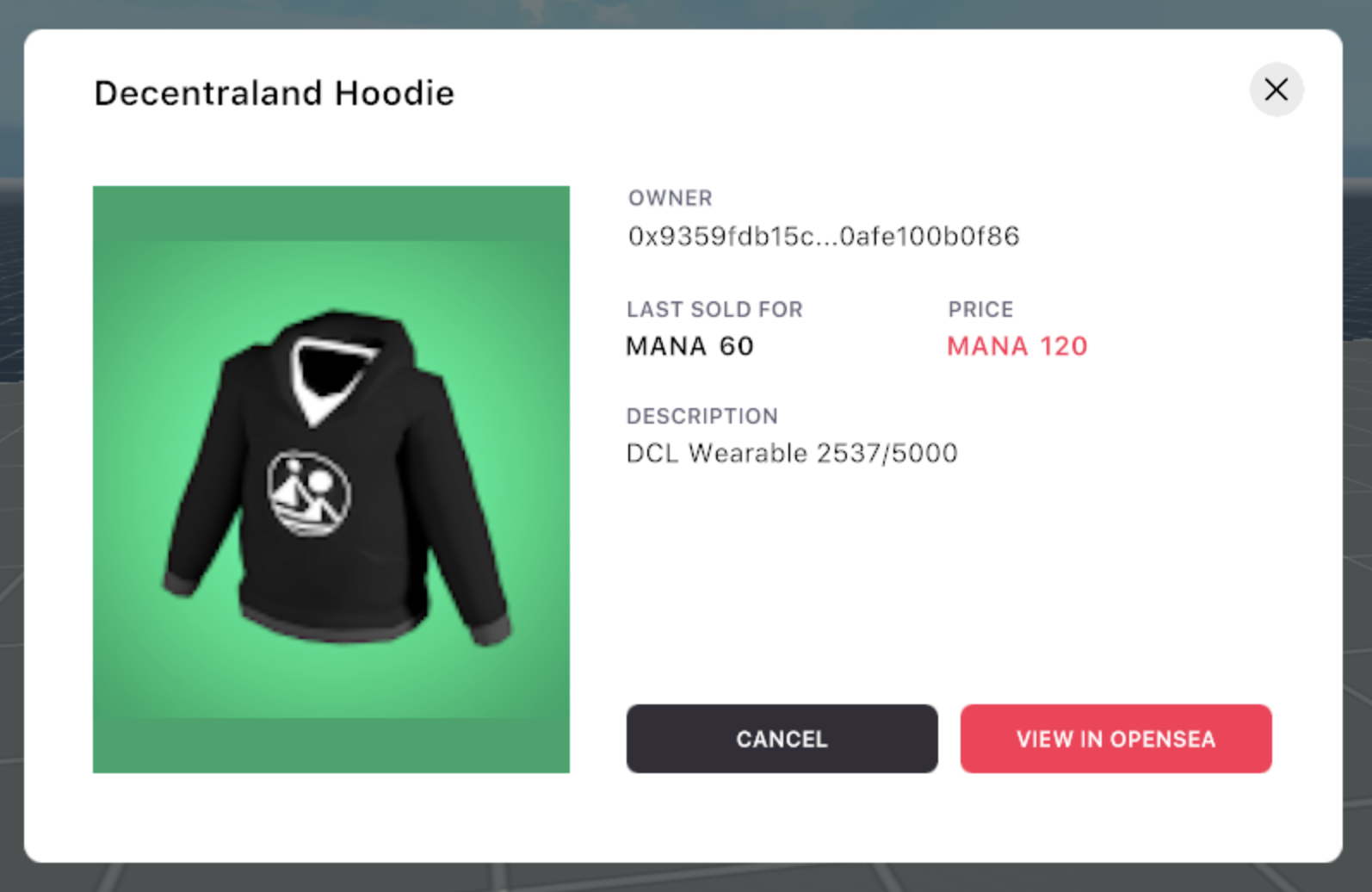
Open this UI by calling the function `openNFTDialog(), passing it the NFT’s contract and id, just like with the NFT shape. The UI must be opened as a result of a button event, to prevent abusive spamming. The button event doesn’t necessarily need to be on the same picture frame or on an NFTShape.
To open this UI, add the following:
myPictureFrame.addComponent(
new OnPointerDown((e) => {
openNFTDialog(
'ethereum://0x06012c8cf97BEaD5deAe237070F9587f8E7A266d/558536'
)
})
)
The UI will include the description that’s available on the NFT, you can also include additional custom text to the UI. To add custom text, simply include the text as a second argument on the openNFTDialog()
function.
myPictureFrame.addComponent(
new OnPointerDown((e) => {
openNFTDialog(
'ethereum://0x06012c8cf97BEaD5deAe237070F9587f8E7A266d/558536',
"This NFT is mine and I'm really proud of owning it."
)
})
)